Understanding PWA Installation
What is a PWA?
A Progressive Web App (PWA) is a web application that can be installed on your device like a native app. PWAs can be installed directly from the browser without going through an app store.
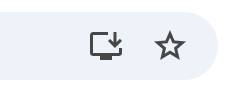
PWA app icon on device
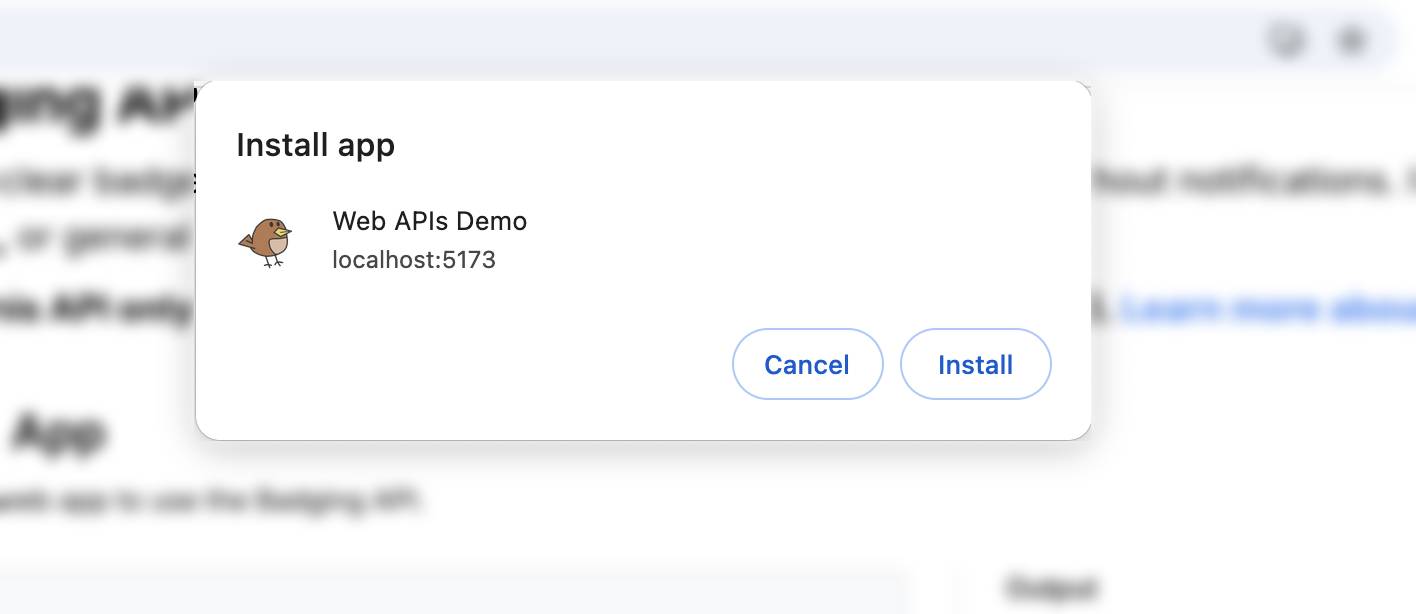
Installation prompt popup
Experimental API
The installation API is experimental and may not work in all browsers. It's best supported in Chromium-based browsers (Chrome, Edge, Opera).
Key Requirements
1. Event Listener Setup
The beforeinstallprompt
event listener must be added at the root level of your application.
let deferredPrompt;
window.addEventListener('beforeinstallprompt', (e) => {
e.preventDefault();
console.log('beforeinstallprompt event captured');
deferredPrompt = e;
});
async function install() {
if (!deferredPrompt) {
console.log('App is already installed or installation not available');
return;
}
deferredPrompt.prompt();
const { outcome } = await deferredPrompt.userChoice;
if (outcome === 'accepted') {
deferredPrompt = null;
}
}
2. Web App Manifest
A valid manifest.json file is required. It tells the browser about your app and how it should behave when installed. Here's our sample manifest:
{
"name": "Web APIs Demo",
"short_name": "Web APIs",
"description": "Explore and test various Web APIs including the Badging API",
"start_url": "/api/badging",
"display": "standalone",
"background_color": "#ffffff",
"theme_color": "#000000",
"id": "/",
"icons": [
{
"sizes": "512x512",
"src": "./web.png",
"type": "image/png"
}
]
}
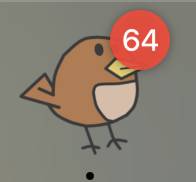
Example of a PWA with badge notification
Link it in your HTML head:
<link rel="manifest" href="/manifest.json" />
Important Notes
- Already Installed Apps: The
beforeinstallprompt
event won't fire if the app is already installed. - Installation Criteria: Browsers have their own criteria for when to allow installation. These typically include:
- Having a valid manifest with required fields
- Being served over HTTPS (or localhost)
- Having appropriate icons defined
Now that you understand PWA installation, head back to the Badging API to try it out with an installed PWA.